Used to execute custom button trigger logic
Example: The user clicks the extension button under the customer, and the interface pops up, and enters the application extension time to trigger approval. After passing, the corresponding high seas recovery time can be delayed.
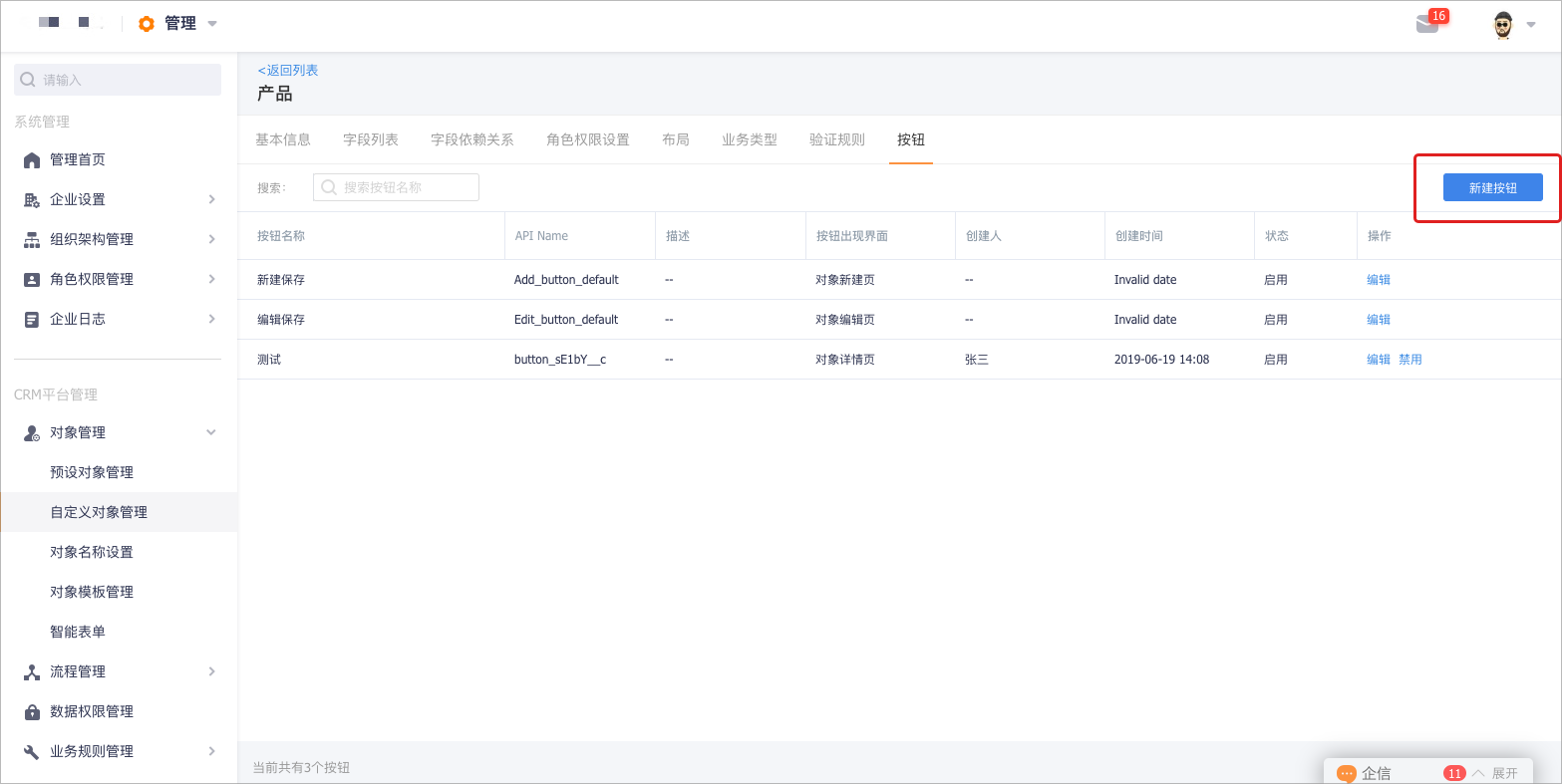
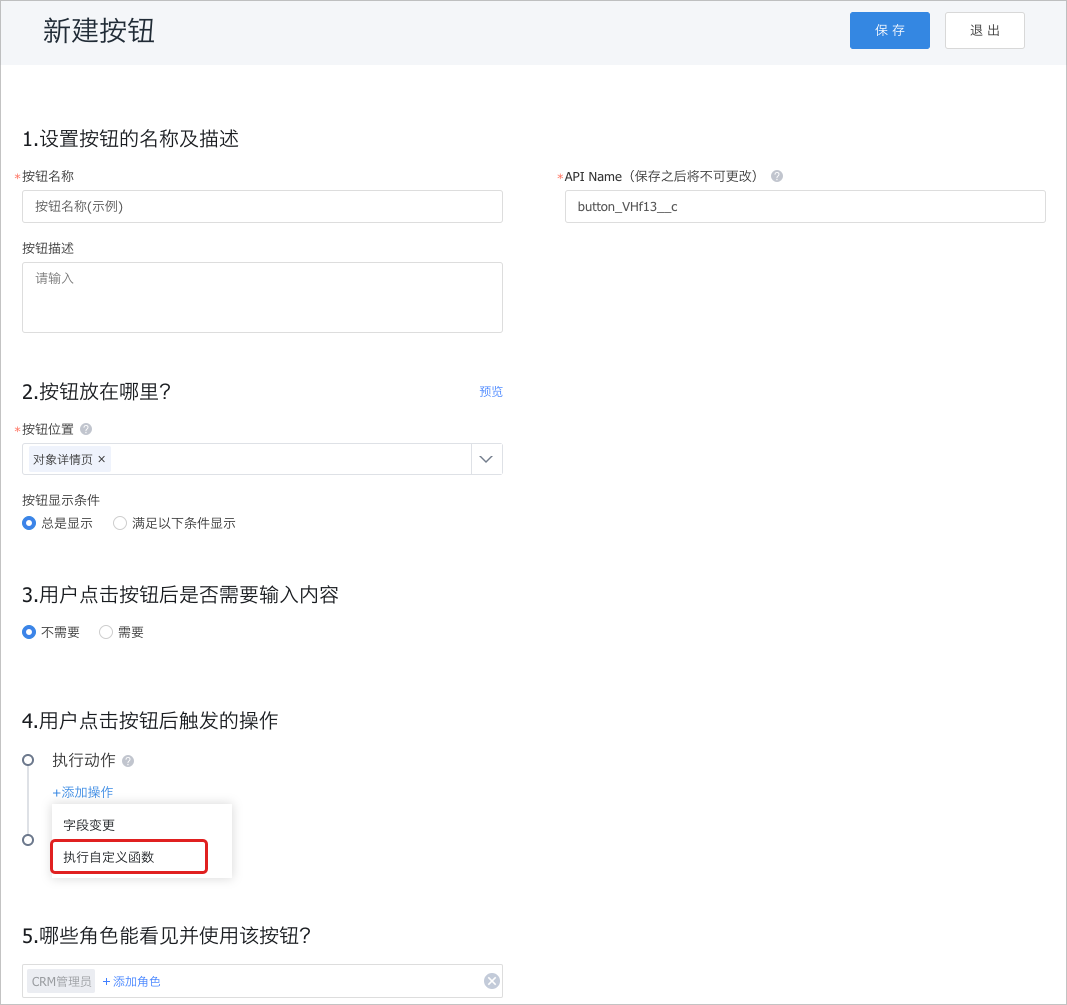
1. Application scenarios of three return types of button functions
1.1 Map
The return value type is Map , which is usually used interactively with the page
Usually the returned information needs to contain
error : Is there an error
errorMessage : The error message prompted after an error occurs
block : Whether to block saving after prompting the exception information
[
"error": true,
"errorMessage": "If it fails, the error message to be prompted",
"block": true
]
1.2 String
Returns a String type, and the content must be a valid URL. After clicking the button, the page jumps to the URL corresponding to the return value.
1.3 UI Actions
In the scenario where the button jumps to a standard page or a custom component, you need to configure a button function whose return type is UIAction.
UIAction jumps to the details page custom component:
Groovy:
UIAction openDialogAction = OpenDialogAction. build{
userData = [:] //List of data key-value pairs passed to the custom component
title = "title" //Title
width = 123 //width and height
maxHeight = 123 //Maximum height
component { // the apiName of the component
apiName = "comp_yuio8__c"
}
}
return openDialogAction
The context.data and context.details of the function will be passed to the custom component as the default parameters objectData and details.
UIAction Jump to list page custom component:
Groovy:
UIAction openDialogAction = OpenDialogAction. build{
userData = [:] //List of data key-value pairs passed to the custom component
title = "title" //Title
width = 123 //width and height
maxHeight = 123 //Maximum height
component { // the apiName of the component
apiName = "comp_yuio8__c"
}
}
return openDialogAction
Java:
import java.util.List;
import java.util.Map;
public class ButtonJava implements IButtonUIAction {
/**
* UIAction jumps to the list page custom component
*/
@Override
public UIAction execute(FunctionContext context, Map<String, Object> args) {
OpenDialogAction.Builder builder = OpenDialogAction.Builder.create();
OpenDialogAction. Component component = OpenDialogAction. Component. create();
component.setApiName("comp_yuio8__c");
Map paraMap = Maps. newHashMap();
paraMap. put("a", 1);
paraMap. put("b", 2);
builder.data = paraMap;//list of data key-value pairs passed to custom components
builder.setTitle("title");//Title
builder.setWidth("123");//width and height
builder.setMaxHeight("123");//maximum heightbuilder.component = component;//apiName of the component
builder.toOpenDialogAction();
return builder.toOpenDialogAction();
}
}
The conext.dataList of the function will be passed to the custom component as the default parameter objectIds.
UIAction jumps to standard components:
Groovy:
//1 Jump to print template
/**
* data Parameters that need to be passed when printing
*/
Map data = [
"validatePreAction": false, //Pre-validation must be false
"templateId":"5e994bbfa5083d97d6ae7afa", //Specify the print template
"dataId":"5d428ed922381800018d946c", //data id
"orientation": "Landscape", //horizontal or vertical
"skipCheckButtonConditions": false // Whether to skip the button display condition judgment of the object
]
WebAction action = WebAction. builder()
.type('print')
.data(data)
.build()
return action
//2 Jump to the new page
Map data = [
'apiname': 'AccountObj',
'record_type': 'default__c'
]
WebAction action = WebAction. builder()
.type('form')
.data(data)
.build()
return action
//3 Jump to the new page and set the default value for the new page field
Map recordData = [
'field_62AcX__c': "6066ec21fe5dbf0024f67e02", //Assign a value for the search associated field
'field_62AcX__c__r': "My product 1", //This method must be used to add the main attribute of the associated object data
'name': 'backfill'
]
Map data = [
'apiname': 'object_qe3x1__c',
'record_type': 'default__c',
'data': recordData,
'details': [:]
]
WebAction action = WebAction. builder()
.type('form')
.data(data)
.build()
return action
//4 Jump to URL
UIAction uiAction = WebAction. builder()
.type('url')
.url('www.fxieoke.com')
.build()
return uiAction
Java:
import java.util.List;
import java.util.Map;
public class UIActionPrint implements IButtonUIAction {
/**
* Jump to print template
*/
@Override
public UIAction execute(FunctionContext context, Map<String, Object> args) {
Map data = Maps.newHashMap(); //data is the parameter that needs to be passed when printing
data.put("validatePreAction", false); //Pre-validation must be false
data.put("templateId", "5e994bbfa5083d97d6ae7afa"); //Specify the print template
data.put("dataId", "5d428ed922381800018d946c"); //data id
data.put("orientation", "Landscape"); //Print horizontally or vertically
data. put("skipCheckButtonConditions", false); //Whether to skip the object's button display condition judgment
UIAction action = WebAction. builder()
.type("print")
.data(data)
.build();
return action;
}
}
import java.util.List;
import java.util.Map;
public class UIActionJump implements IButtonUIAction {
/**
* Jump to the new object page
*/
@Override
public UIAction execute(FunctionContext context, Map<String, Object> args) {
Map data = Maps.newHashMap(); //data object jump parameters
data.put("apiname", "AccountObj"); //Object API name
data.put("record_type", "default__c"); //object business type
UIAction action = WebAction. builder()
.type("form")
.data(data)
.build();
return action;
}
}
import java.util.List;
import java.util.Map;
public class UIActionJumpDefault implements IButtonUIAction {
/**
* Jump to the new page and set the default value for the new page field
*/
@Override
public UIAction execute(FunctionContext context, Map<String, Object> args) {
Map recordData = Maps. newHashMap();
recordData.put("field_62AcX__c", "6066ec21fe5dbf0024f67e02"); //Assign values for search associated fields
recordData.put("field_62AcX__c__r", "My product 1"); //This method must be used to add the main attribute of the associated object data
Map details = Maps. newHashMap();
Map data = Maps.newHashMap(); //data object jump parameters
data.put("apiname", "object_qe3x1__c"); //Object API name
data.put("record_type", "default__c"); //object business type
data.put("data", recordData); //object business type
data.put("detais", detais); //object business type
UIAction action = WebAction. builder()
.type("form")
.data(data)
.build();
return action;
}
}
import java.util.List;
import java.util.Map;
public class UIActionJumpURL implements IButtonUIAction {
/**
* Jump to URL
*/
@Override
public UIAction execute(FunctionContext context, Map<String, Object> args) {
UIAction action = WebAction. builder()
.type("url")
.url("www.fxieoke.com")
.build();
return action;
}
}
**UIAction The mobile terminal returns pop-up window information: **
Groovy:
AlertAction action = AlertAction. builder()
.type('default')
.text('Prompt information')
.build()
return action
//Prompt information cannot be empty, type is to reserve the style attributes of various pop-up box prompts later, it can be empty, empty or default inserted into default
Java:
import java.util.List;
import java.util.Map;
public class UIActionMessage implements IButtonUIAction {
/**
* The mobile terminal returns pop-up information
*/
@Override
public UIAction execute(FunctionContext context, Map<String, Object> args) {
//Prompt information cannot be empty, type is to reserve the style attributes of various pop-up box prompts later, it can be empty, empty or default inserted into default
AlertAction action = AlertAction. builder()
.type("default")
.text("prompt information")
.build();
return action;
}
}
UIAction mobile jump page:
Groovy:
AppAction app = AppAction.builder()
.url('https://www.baidu.com')
.build()
return app
Java:
import java.util.List;
import java.util.Map;
public class UIActionPhoneJump implements IButtonUIAction {
/**
* Mobile terminal jump page
*/
@Override
public UIAction execute(FunctionContext context, Map<String, Object> args) {
AppAction action = AppAction.builder()
.url("https://www.baidu.com") //The url address of the jump
.build();
return action;
}
}