Used to execute regularly scheduled function tasks
Actual scenario: Regularly process customers in batches, and the customers whose latest follow-up time is earlier than half a year ago are invalidated
Configuration method: [Management] - [Customized Development Platform] - [Planning Task]
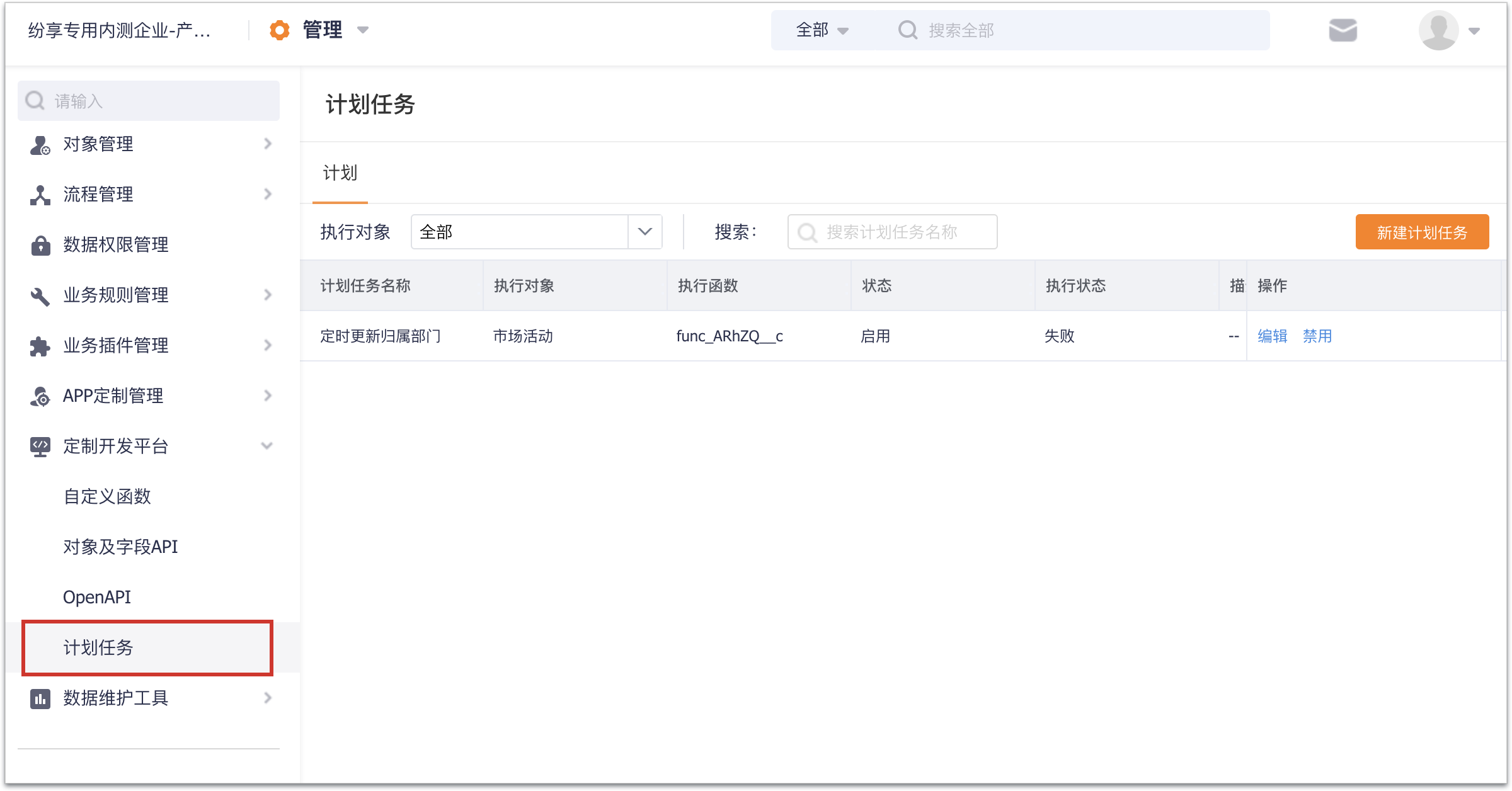
Execution object: For the object type that needs to be processed in batches, each scheduled task can only bind one object
execution function: the processing logic that needs to be executed
Execution Conditions: According to the fields of the execution object, filter out the object instances that need to be processed (execution function)
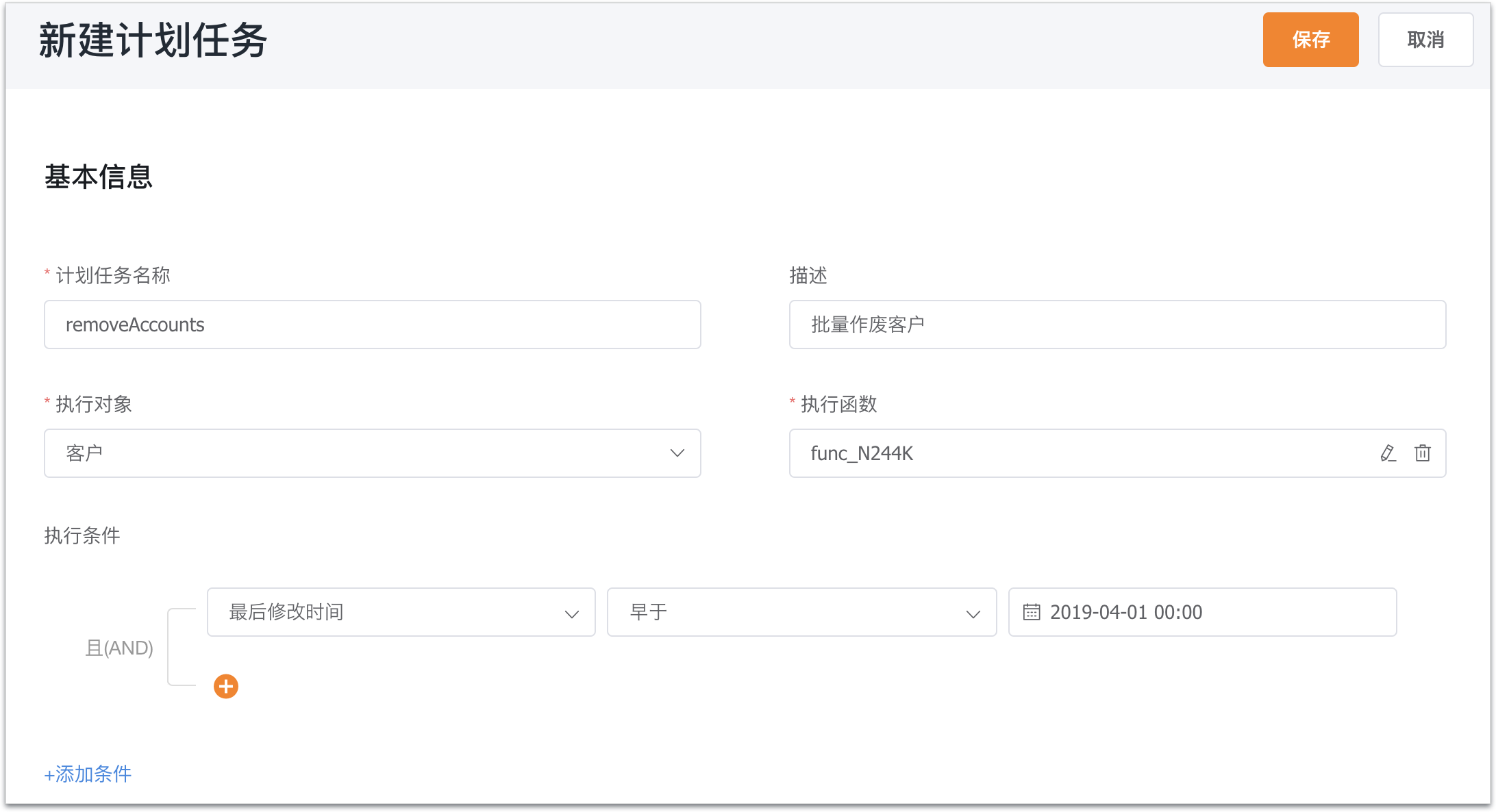
Write a function template:
//Eligible data id
List ids = context. objectIds as List
def(boolean error,List dataList,String errorMessage) = Fx.object.findByIds("NewOpportunityObj",ids)
dataList. each { item ->
Map map = item as Map
//Process business logic
Fx.log.info(map.name)
}
Cron expression:
The execution time of scheduled tasks can be flexibly configured using Cron expressions.
Rules for filling in each time field:
| Name | Values | Special Characters |
| :---------------- | :------------------------ | :--- -------------- |
| Seconds | 0–59 | None |
| Minutes | 0–59 | None |
| Hours | 0–23 | , - * / |
| Day of Month | 1–31 | , - * ? / L W |
| Month | 1–12 or JAN-DEC | , - * / |
| Day of Week | 1–7 or SUN-SAT (1=SUN) | , - * ? / L # |
| year (optional) (Year) | empty or 1970–2099 | , - * / |
Each field uses numbers, but the following special characters can also appear, and their meanings are:
(1) *: Indicates to match any value of the domain. If * is used in the Hours field, it means that the event will be triggered every hour.
(2)?: No specific value specified. Applicable only for DayofMonth and DayofWeek, after setting a specific value for one of these two time fields, the other must be set to ? .
(3) -: Indicates the range. For example, use 5-20 in the Hours field, indicating that it is triggered every hour from 5 o'clock to 20 o'clock
(4) /: Indicates that the trigger starts at the starting time, and then triggers every fixed time. For example, if 1/5 is used in the DayofMonth field, it means that it is triggered every 5 days, and the first trigger is the 1st.
(5),: Indicates to list enumeration values. For example: use 5,20 in the Hours field, it means trigger once at 5 o'clock and 20 o'clock respectively.
(6) L: Indicates the last, it can only appear in the fields of DayofWeek and DayofMonth. If 5L is used in the DayofWeek field, it means that it will be triggered on the last Thursday.
(7) W: Indicates a valid working day (Monday to Friday), which can only appear in the DayofMonth field, and the system will trigger the event on the nearest valid working day to the specified date. For example: use 5W on DayofMonth, if the 5th is a Saturday, it will be triggered on the nearest working day: Friday, which is the 4th. If the 5th is Sunday, it will be triggered on the 6th (Monday); if the 5th falls on a day from Monday to Friday, it will be triggered on the 5th. Another point, W's most recent lookup does not span months.
(8) LW: These two characters can be used together to indicate the last working day of a certain month, that is, the last Friday.
(9) #: It is used to determine the day of the week of each month, and it can only appear in the DayofMonth field. For example, in 4#2, it means the second Wednesday of a certain month.
Examples of common expressions:
(1) "0 0 2 1 * ? *" means to adjust the task at 2 am on the 1st of each month
(2) "0 15 10 ? * MON-FRI" indicates that the job is executed at 10:15 every morning from Monday to Friday
(3) "0 15 10 ? 6L 2002-2006" indicates that the operation will be executed at 10:15 am on the last Friday of each month from 2002 to 2006
(4) "0 0 10,14,16 * * ?" 10 am, 2 pm, 4 pm every day
(5) "0 0 12 ? * WED" means every Wednesday at 12 noon
(6) "0 0 12 * * ?" Triggered at 12 noon every day
(7) "0 15 10 ? * *" is triggered every morning at 10:15
(8) "0 15 10 * * ?" is triggered every morning at 10:15
(9) "0 15 10 * * ? *" is triggered every morning at 10:15
(10) "0 15 10 * * ? 2005" Triggered at 10:15 am every day in 2005
(11) "0 10,44 14 ? 3 WED" Triggered at 2:10 and 2:44 pm on Wednesdays in March every year
(12) "0 15 10 ? * MON-FRI" is triggered at 10:15 am from Monday to Friday
(13) "0 15 10 15 * ?" Triggered at 10:15 am on the 15th of each month
(14) "0 15 10 L * ?" Triggered at 10:15 am on the last day of each month
(15) "0 15 10 ? * 6L" Triggered at 10:15 am on the last Friday of each month
(16) "0 15 10 ? * 6L 2002-2005" Triggered at 10:15 am on the last Friday of each month from 2002 to 2005
(17) "0 15 10 ? * 6#3" Triggered at 10:15 am on the third Friday of each month