Used to update/verify data and backfill the interface when a change occurs on a new/edit page
Configuration method: In the layout of object management, find UI events in the global settings on the right
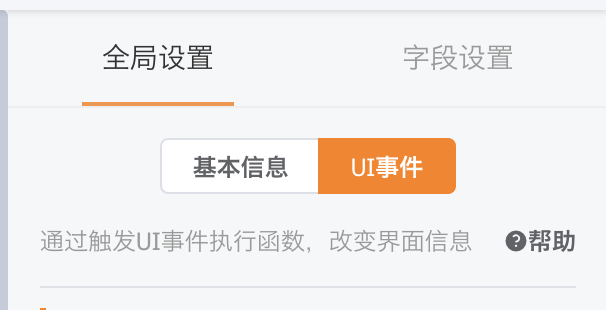
Note:
- The slave object does not support triggering UI events
2. The execution result of the UI event is only applied to the new/edit page, and the actual effect depends on whether it is saved or not
3. All custom objects support UI events, and some preset objects support (sales leads, customers, orders/order products, business opportunities 2.0/business opportunities 2.0 details)
4. Only the flagship version and group version support this ability
1. Data update event
- On the new/edit page, when a field is modified (value changes and out of focus), trigger a custom function to update the data of the master object or slave object
Actual scenario: When creating a new order, after selecting a customer, the customer's address can be directly synchronized to the delivery address field of the order
Configuration method: UI event - add data update event - classify selection field event
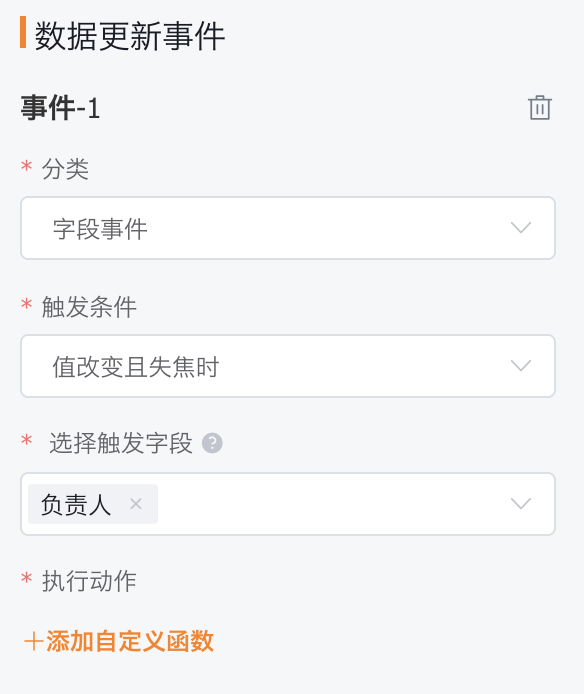
Function writing template:
Groovy:
//New UIEvent event
UIEvent event = UIEvent. build(context) {
//Set the field to read-only, hidden
editMasterFields "field_I18ri__c" readOnly(true) hidden(true)
//Set reminder message
reminder Remind.Text("Pop-up window reminder")
//main object modify data
editMaster("a": 1, "b": 2)
//Add a slave object, to add a slave object, the business type must be specified, and it is the business type displayed in the current layout
//If the business type does not match. The slave object cannot be added
addDetail "detailApiName" set("a": 1, "b": 2)
//Delete the slave object according to the condition, delete the slave object that returns true in where
removeDetail "detailApiName" where { x -> (x["a"] as Integer) > 0 }
// Edit the slave object according to the condition and the same reason as above will only process the slave object data that returns true in where
editDetail "detailApiName" set("a": 1, "b": 2) where { x -> (x["a"] as Integer) > 0 }
//removeDetail and editDetail can not add where so that it will directly affect all data
//The contents of set and editMaster must be in the form of map or key:valued
removeDetail "detailApiName"
editDetail "detailApiName" set("a": 1, "b": 2)
// Hide the secondary object whose apiName is object_0uyAd__c (Note: After the secondary object is hidden, the hidden secondary object data will be cleared when saving data, remember to use it with caution!!)
editObject 'object_0uyAd__c' hidden(true)
// hide the business type from the object
editDetailRecordType "object_qep6N__c" recordType("defaultA__c") hidden(true)
// option value hidden
editOption "object_qep6N__c" fieldApiName("field_y210t__c") option("wwxJqgnO4") hidden(true)
// hide batch edit button field_y1XV1__c
editDetailButton "object_qep6N__c" recordType("defaultA__c") hiddenButton(ButtonAction.BATCH_UPDATE, true)
// hide bulk delete button
editDetailButton "object_qep6N__c" recordType("defaultA__c") hiddenButton(ButtonAction.BATCH_DELETE, true)
// hide the bulk copy button
editDetailButton "object_qep6N__c" recordType("defaultA__c") hiddenButton(ButtonAction.BATCH_CLONE, true)
// hide the common add row button
editDetailButton "object_qep6N__c" recordType("defaultA__c") hiddenButton(ButtonAction. SINGLE_ADD_ONE, true)
// Hide common button -- find association from object
editDetailButton "object_qep6N__c" recordType("defaultA__c") lookupFieldApiName("field_y1XV1__c") hiddenButton(ButtonAction.BATCH_LOOKUP_CREATE, true)
// hide single delete operation
editDetailButton "object_qep6N__c" recordType("defaultA__c") hiddenButton(ButtonAction. SINGLE_DELETE, true) where { x -> (x["a"] as Integer) > 0 }
// hide single copy operation
editDetailButton "object_qep6N__c" recordType("defaultA__c") hiddenButton(ButtonAction. SINGLE_CLONE, true) where { x -> (x["a"] as Integer) > 0 }
// readonly hide from object fields
editDetailFields "object_qep6N__c" fieldApiName("field_jt9F4__c") hidden(true) readOnly(true)
editDetailFields "object_qep6N__c" fieldApiName("field_y220t__c") hidden(true) readOnly(true)
// Obtain the deleted slave object method 1
def deletedDetails = (context.arg as Map)["deletedDetails"] as Map
UIEvent event = UIEvent.build(context){
}
// Obtain the deleted slave object method 2
Map currentDeletedDetail = event.getCurrentDeletedDetail()
}
return event
Java:
import java.util.List;
import java.util.Map;
import com.fxiaoke.functions.UIEvent;
public class UIEventCode implements IUIEventAction {
/**
* The running method of the UI event function
*/
@Override
public UIEvent execute(FunctionContext context, Map<String, Object> args) {
UIEvent.Builder builder = UIEvent.Builder.create(context);
//Set the field to read-only, hidden
builder.editMasterFields("field_I18ri__c").readOnly(true).hidden(true);
//Set reminder message
builder.remind(Remind.Text("Pop-up reminder"));
//main object modify data
builder.editMaster(Maps.of("a", 1, "b", 2));
//Add a slave object, to add a slave object, the business type must be specified, and it is the business type displayed in the current layout
//If the business type does not match. The slave object cannot be added
builder.addDetail("detailApiName").set(Maps.of("a", 1, "b", 2));
//Delete the slave object according to the condition, delete the slave object that returns true in where
builder.removeDetail("detailApiName").where(x -> ((Integer) x.get("a")) > 0);
// Edit the slave object according to the condition and the same as above, only the slave object data returned as true in where will be processed, and the where method uses lambda expression
builder.editDetail("detailApiName").set(Maps.of("a", 1, "b", 2)).where(x -> ((Integer) x.get("a")) > 0) ;
//removeDetail and editDetail can not add where so that it will directly affect all data
//The contents of set and editMaster must be in the form of map or key:valued
builder. removeDetail("detailApiName");
builder.editDetail("detailApiName").set(Maps.of("a", 1, "b", 2));
// hide the apiName as object_0uyAd__c from the object
builder.editObject("object_0uyAd__c").hidden(true);
UIEvent event = builder. getUIEvent();
return event;
}
}
- Create/edit pages at the same time as the master and slave. When creating/editing/deleting a slave object, trigger a custom function to update the data of the master object/slave object (provided that the object has a slave object, there will be a slave object event entry )
Actual scenario: For each new order product detail, assign a value to the discount amount field of the product detail according to the customer level
Configuration method: UI event - Add data update event - Classification selection from object event, trigger condition can choose to add details/edit details/delete details
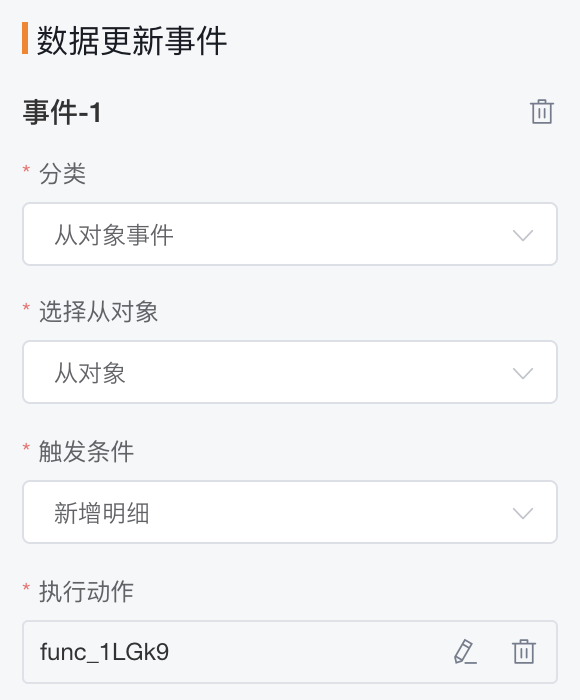
Function writing template:
Groovy:
UIEvent event = UIEvent. build(context) {
//Master object, slave object data modification, see above for details
}
// Get the slave object data of the current operation
Map currentData = event. getCurrentDetail()
//Modify the slave object data of the current operation (mainly used in the scenarios of creating and editing details)
currentData.put("From the ApiName of the object field", "The value of this field needs to be changed")
currentData.put("ApiName from object field 1", "The value of this field 1 needs to be changed")
//Get the currently added slave object data
List currentData = event. getCurrentAddDetail()
return event
Java:
import java.util.List;
import java.util.Map;
import com.fxiaoke.functions.UIEvent;
public class UIEventCode implements IUIEventAction {
/**
* The running method of the UI event function
*/
@Override
public UIEvent execute(FunctionContext context, Map<String, Object> args) {
UIEvent.Builder builder = UIEvent.Builder.create(context);
UIEvent event = builder. getUIEvent();
// Get the slave object data of the current operation
Map currentData = event. getCurrentDetail();
//Modify the slave object data of the current operation (mainly used in the scenarios of creating and editing details)
currentData.put("From the ApiName of the object field", "The value of this field needs to be changed");
currentData.put("ApiName from object field 1", "The value of this field 1 needs to be changed");
//Get the currently added slave object data
List list = event. getCurrentAddDetail();
return event;
}
}
- Return error information to the page example:
Fx.message.throwErrorMessage("error message");
return null;
2. Page load event
On the new/edit page, when the page loads, trigger a custom function to update the data of the master object or the slave object
Actual scenario: When creating an electronic signature, automatically create the signer's slave data based on the business data associated with the electronic signature
Configuration method: UI event - add data update event - category selection page load event
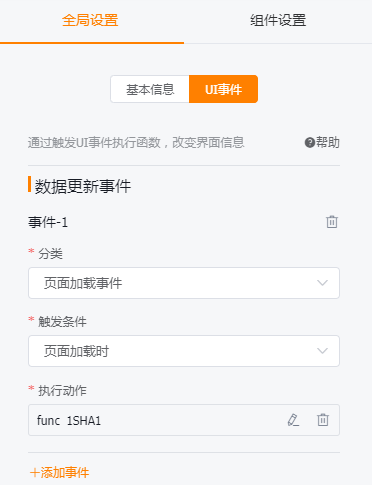
Function writing template: reference data update event
3. Check event
On the new/edit page, when a field is modified (value changes and out of focus), trigger a custom function to verify whether the field value meets a specific condition
Actual scenario: When filling in the wrong format of the mobile phone number/email, the front-end prompt can be given immediately
Configuration method: UI event - add verification event
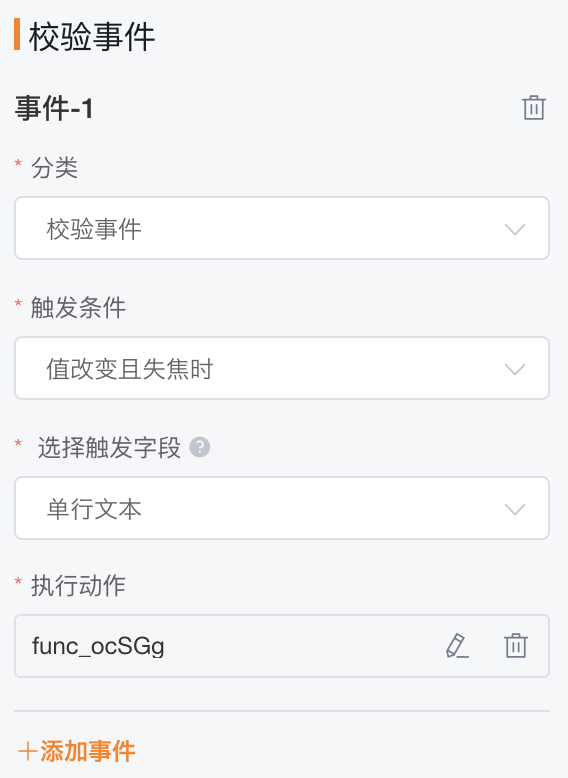
Function writing template:
Groovy:
//Red text reminder
Remind remind = Remind. Text("Text");
// popup reminder
Remind remind = Remind. Alert("Text");
//Set reminder message
remind Remind. builder()
.remindText("name", "The name of the main attribute is repeated")
.remindText("field_y2k46__c", "Field cannot contain test")
.build()
//Clear the reminder message
remind Remind. builder()
.remindText("name", "")
.remindText("field_y2k46__c", "")
.build()
return remind;