You can define classes, write custom classes and custom functions with the idea of object-oriented programming, and the methods of the written APL class can be called by other functions
1. Configuration method
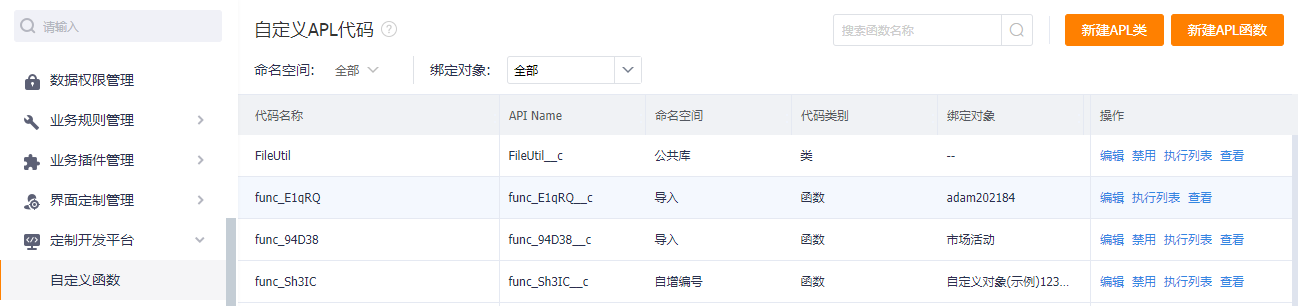
Note: 1. When naming a class, start with a capital letter, allow alphanumeric characters in the middle, must end with __c, and cannot exceed 50 characters
2. The class name of the APL class is the apiName of the function except the part of __c, which cannot be modified
3. Objects cannot be instantiated directly through the new keyword in the function. Add a Fx.klass.newInstance to instantiate the APL class, Object object = Fx.klass.newInstance(String str)
4. The static method can be called directly through the class name + method name without instantiation; while the ordinary method needs to be instantiated before it can be called, and the object is instantiated through Fx.klass.newInstance
- When writing APL classes, you can debug through the main function
2. Function writing template
Groovy:
class HtttpUtils {
private static String CONYTENT_TYPE = 'Content-Type'
private static String JSON = 'application/json'
private static String MULTIPART = 'multipart/form-data'
//application/json post request
public static Map post(String url, Map header, Map body) {
header.put(CONYTENT_TYPE, JSON)
def (Boolean error, HttpResult data, String errorMessage) = Fx.http.post(url, header, body)
if (error) {
log. info(errorMessage)
return [:]
}
log.info('HtttpUtils execute success!')
return data['content']
}
//multipart/form-data request, byte file upload
public Map post(String url, byte[] data, String name, String filename, String contentType) {
String boundary = Fx.random.randomUUID()
Map header = [
CONYTENT_TYPE: MULTIPART + '; boundary=' + boundary
]
boundary = '--' + boundary
String param = boundary + '\n' +
'Content-Disposition: form-data; name=' + name + '; filename=' + filename +'\n' +
'Content-Type: ' + contentType + '\n' +
'\n' +
data + '\n' +
boundary + '--\n'
log. info(param)
def (Boolean error, HttpResult ret, String errorMessage) = Fx.http.post(url, header, param)
if (error) {
log. info(errorMessage)
return [:]
}
log.info('HtttpUtils execute success!')
return ret['content']
}
public static void main(String[] args) {
String url = "http://httpbin.org/post"
Map body = ["key": "value"]
Map header = [:]
// The static method can be called directly through the class name + method name without instantiation
Map ret = HtttpUtils. post(url, header, body);
log. info(ret)String name = 'file'
String filename = 'test.png'
byte[] data = [1, 2, 3]
String contentType = 'image/png'
// Ordinary methods need to be instantiated before they can be called
// Need to instantiate the object through Fx.klass.newInstance
HtttpUtils utils = Fx.klass.newInstance('HtttpUtils')
Map ret1 = utils. post(url, data, name, filename, contentType);
log.info(ret1)
}
}
Java:
import java.util.List;
import java.util.Map;
public class JavaUtil {
private String CONYTENT_TYPE = "Content-Type";
private String JSON = "application/json";
private String MULTIPART = "multipart/form-data";
//application/json post request
public Map post(String url, Map header, Map body) {
header.put(CONYTENT_TYPE, JSON);
APIResult data = Fx.http.post(url, header, body);
if ((boolean)data.get(0)) {
log.info(data.get(2));
return null;
}
Fx.log.info("HtttpUtils execute success!");
HttpResult result = (HttpResult)data.get(1);
return (Map) result. getContent();
}
//multipart/form-data request, byte file upload
public Map post(String url, byte[] data, String name, String filename, String contentType) {
String boundary = Fx. random. randomUUID();
Map header = Maps. newHashMap();
header.put(CONYTENT_TYPE, JSON);
boundary = "--" + boundary;
String param = boundary + "\n" +
"Content-Disposition: form-data; name=" + name + "; filename=" + filename +"\n" +
"Content-Type: " + contentType + "\n" +
"\n" +
data + "\n" +
boundary + "--\n";
Fx.log.info(param);
APIResult apiResult = Fx.http.post(url, header, param);
if ((boolean)apiResult. get(0)) {
log.info(apiResult.get(2));
return null;
}
Fx.log.info("HtttpUtils execute success!");
HttpResult result = (HttpResult)apiResult.get(1);
return (Map) result. getContent();
}
//Function debugging method
public void debug() {
String url = "http://httpbin.org/post";
Map body = Maps.of("key", "value");
Map header = Maps. newHashMap();
// The static method can be called directly through the class name + method name without instantiation
Map data = post(url, header, body);
Fx.log.info(data);
String name = "file";
String filename = "test.png";
byte[] data1 = new byte[]{1, 2, 3};
String contentType = "image/png";
// Ordinary methods need to be instantiated before they can be called
// Need to instantiate the object through Fx.klass.newInstance
JavaUtil utils = Fx.klass.newInstance(JavaUtil.class);
Map ret1 = utils. post(url, data1, name, filename, contentType);
log.info(ret1);
}
}