1. Verify the APL code before importing
Function validation for data before import
1.1 Configuration method
In the import settings of object management, click Verify APL code before adding
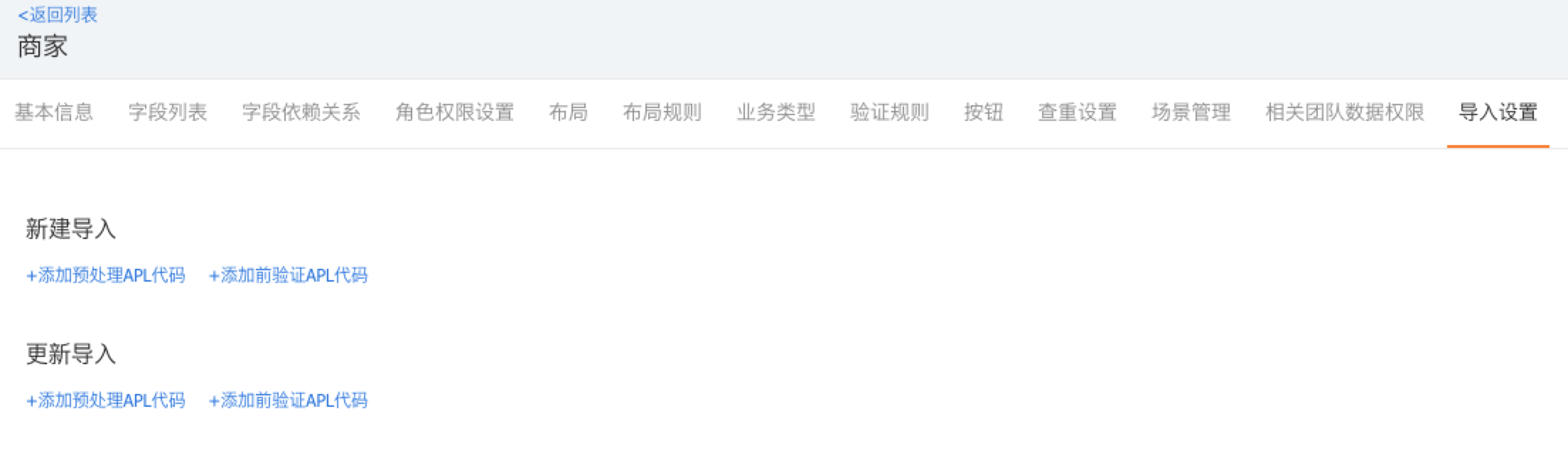
Note: 1. The pre-import verification function is similar to the newly-created and edited pre-verification function, which can prompt verification information and support blocking;
-
If the data import fails, or if there is an error in the verification, the error information will be backfilled into the Excel failure list
-
The return value type is validateResult
1.2 Function writing template:
Groovy:
// check logic
ValidateResult validate = ValidateResult. builder()
.success(false)
.errorMessage("error message")
.build()
return validate
Java:
import java.util.List;
import java.util.Map;
public class Import implements ImportAction {
/**
* How to import the function
*/
@Override
public ValidateResult execute(FunctionContext context, Map<String, Object> args) {
// check logic
ValidateResult validate = ValidateResult. builder()
.success(false)
.errorMessage("error message")
.build();
return validate;
}
}
2. Import preprocessing APL code
It is used to preload all imported data in batches before importing, and do data preprocessing.
2.1 Configuration method
In the import settings of object management, click Add preprocessing APL code
2.2 Function writing template
Preprocessing function:
Groovy:
// Get the imported task id and and whether it is the last batch of data
def taskId = context.task.taskId as String
log.info(context.task.taskId)
log.info(context.task.lastBatch)
// Cache information in the cache for use by the pre-validation function
Cache cache = Fx.cache.defaultCache
List<Map> dataList = context. dataList as List
dataList. each {data->
def rowNo = data._RowNo as String
def name = data.field_MG1ch__c as String
def key = taskId + "_" + rowNo
log. info(key)
def value = "" + name
cache. put(key, value, 30)
}
return ValidateResult. builder()
// Returning false will terminate the import
.success(false)
.errorMessage("I am importing preprocessing function!!!!")
.build()
Java:
import java.util.List;
import java.util.Map;
public class Import implements ImportAction {
/**
* How to import the function
*/
@Override
public ValidateResult execute(FunctionContext context, Map<String, Object> args) {
// Get the imported task id and and whether it is the last batch of data
String taskId = context.getTask().get("taskId").toString();
Fx.log.info(context.getTask().get("taskId").toString());
Fx.log.info(context.getTask().get("lastBatch").toString());
// Cache information in the cache for use by the pre-validation function
Cache cache = Fx.cache.getDefaultCache();
List dataList = (List) context. getDataList();
for (Object item: dataList) {
Map map =(Map<String, Object>)item;
String rowNo = map. get("_RowNo"). toString();
String name = map. get("name"). toString();
String key = taskId + "_" + rowNo;
Fx.log.info(key);
String value = name;
cache. put(key, value, 30);
}
ValidateResult validate = ValidateResult. builder()
// Returning false will terminate the import
.success(false)
.errorMessage("I am importing preprocessing function!!!!")
.build();
return validate;
}
}
Pre-authentication function:
Groovy:
log. info(context. data)
def data = context.data as Map
// Get the task id and the row number to which the data belongs
def taskId = data._TaskId as String
def rowNo = data._RowNo as String
log. info(taskId)
log. info(rowNo)
// Obtain the information saved by the preprocessing function from the cache
Cache cache = Fx.cache.defaultCache
def key = taskId + "_" + rowNo
def value = cache. get(key)
log. info(value)
return ValidateResult. builder()
.success(true)
.errorMessage("I am a validation function before import!!!!")
.build()
Java:
import java.util.List;
import java.util.Map;
public class BeforeImport implements ImportAction {
/**
* How to import the function
*/
@Override
public ValidateResult execute(FunctionContext context, Map<String, Object> args) {
Fx.log.info(context.getData());
Map data = (Map) context. getData();
// Get the task id and the row number to which the data belongs
String taskId = data.get("_TaskId").toString();
String rowNo = data. get("_RowNo"). toString();
Fx.log.info(taskId);
Fx.log.info(rowNo);
// Obtain the information saved by the preprocessing function from the cache
Cache cache = Fx.cache.getDefaultCache();
String key = taskId + "_" + rowNo;
String value = cache.get(key).toString();
Fx.log.info(value);
ValidateResult validate = ValidateResult. builder()
.success(true)
.errorMessage("I am a validation function before import!!!!")
.build();
return validate;
}
}