It is used to set the selectable range of this field when creating/editing a lookup associated field
Configuration method: When creating or editing a lookup associated field, set the selectable data range to "meet the following conditions" - set the condition - based on a custom function - add a custom function
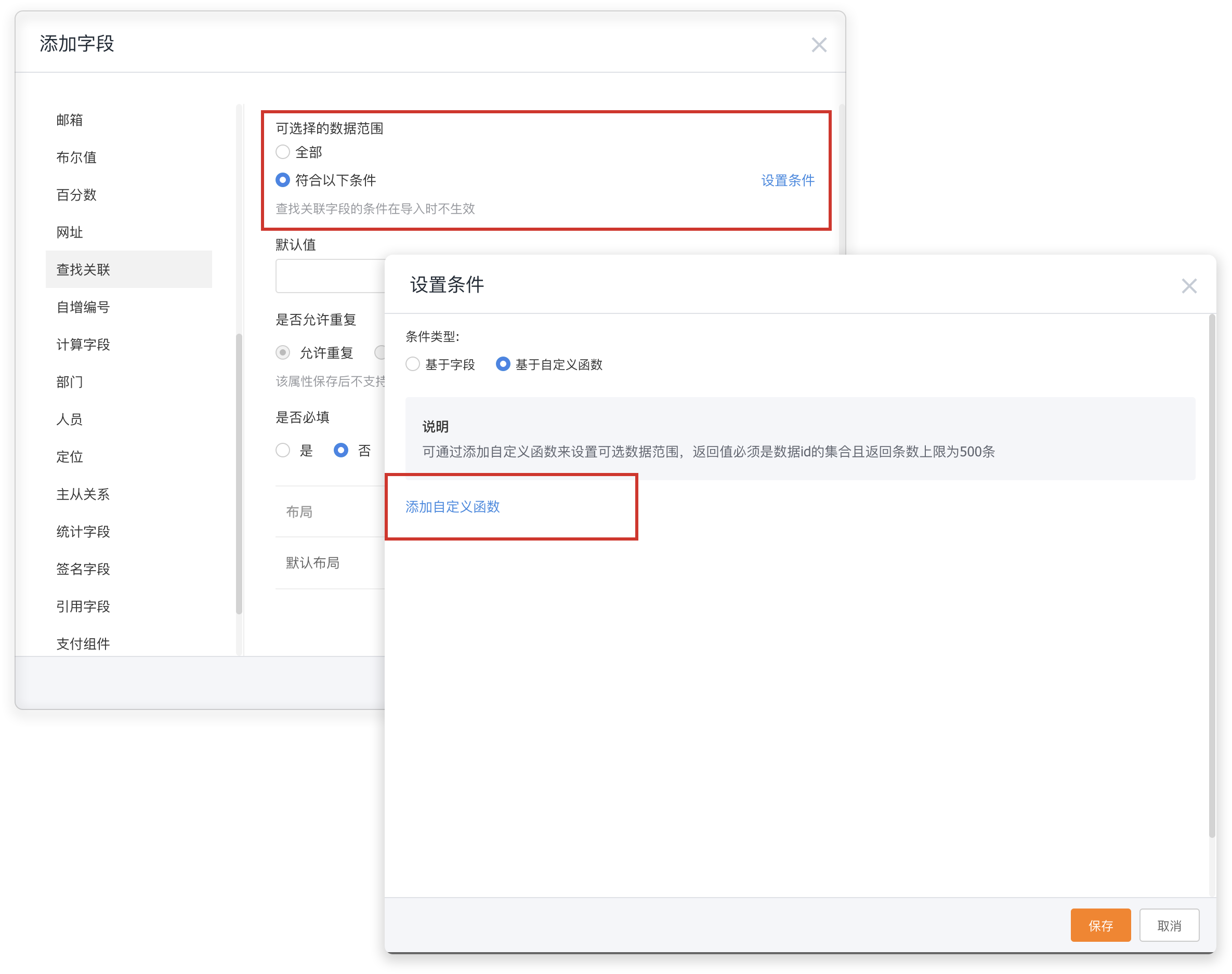
1. The return type is QueryTemplate
QueryTemplate is more efficient as the return result of the conditions of the associated query.
Unless the query method of QueryTemplate cannot meet the requirements, it is preferred to use the QueryTemplate return type function. The range data returned by this method has no upper limit, and the execution efficiency is high.
Function writing template:
Groovy:
QueryTemplate template1 = QueryTemplate. AND(
["name":Operator.LIKE("test")],
["field_g7Zeh__c":Operator.LTE("Test single-line text")]
)
QueryTemplate template2 = QueryTemplate. AND(
["owner":Operator. LIKE("1000")],
["record_type":Operator.EQ("default__c")]
)
//QueryTemplate needs to be opened separately when using the OR condition, please contact the sales staff to place an order to activate the product: [object list filtering support or]
QueryTemplate template3 = QueryTemplate.OR(template1,template2)
the
return template3
// query condition support
// product_id is the search associated field, and the query template supports filtering results that the name field under the associated object meets certain conditions
QueryTemplate template = QueryTemplate. AND(
["product_id.name":Operator.EQ("Test")]
)
return template
Java:
import java.util.List;
import java.util.Map;
public class RangeTemplate implements IQueryTemplateAction {
/**
* The operation method of the range rule function (QueryTemplate)
*/
@Override
public QueryTemplate execute(FunctionContext context, Map<String, Object> args) {
//Construct query conditions
QueryTemplate template1 = QueryTemplate. AND(
Maps.of("name", QueryOperator.EQ("test")),
Maps.of("field_g7Zeh__c", QueryOperator.EQ("Test a single line of text"))
);
QueryTemplate template2 = QueryTemplate. AND(
Maps.of("owner", QueryOperator. LIKE("1000")),
Maps.of("record_type", QueryOperator.EQ("default__c"))
);
//QueryTemplate needs to be opened separately when using the OR condition, please contact the sales staff to place an order to activate the product: [object list filtering support or]
QueryTemplate template3 = QueryTemplate.OR(template1,template2);
return template3;
}
}
2. The return type is set (List)
It is not recommended to use the List return type function. The data returned by this method has an upper limit of 500 items, and the execution efficiency of query and using id as the return value is low.
Function writing template:
//Define id List
List objectIds = []
...
//Write function logic and add optional data Id to objectIds
objectIds. add()
//The final returned data result is List<String> which contains the data id that satisfies the condition
return objectIds
Actual Scenario: The quotation details are restricted by product classification to select the product range, and the quotation details of different business types select different categories of product data.
Template case based on the actual scenario above:
Groovy:
// Get the field value of the current operation object instance
String product = context.data.field_wPnHu__c
//Find data according to conditions, query the required data according to the required business logic
def ret = Fx.object.find("Find the object ApiName corresponding to the associated field", [["field_1tG48__c":product]],100,0)
//If the query is wrong, return directly
if( ret[0] ){
Fx.log.info("query exception")
return []
}
//Define id List
List objectIds = []
QueryResult result = ret[1] as QueryResult
//Loop through the query results and add all Ids to objectIds
result.dataList.each{ item ->
Map map = item as Map
objectIds.add(map._id)
}
//Finally return objectIds
return objectIds
Java:
import java.util.List;
import java.util.Map;
public class RangeList implements IQueryListAction {
/**
* The operation method of the range rule function (List)
*/
@Override
public List execute(FunctionContext context, Map<String, Object> args) {
// Get the field value of the current operation object instance
String name = context.getData().get("name").toString();
Fx.log.info(name);
List<Object> list = Lists. newArrayList();
list.add(Maps.of("name", QueryOperator.EQ(name)));
//Find data according to conditions, query the required data according to the required business logic
APIResult ret = Fx.object.find("AccountObj",list,100,0);
QueryResult result = (QueryResult)ret. getData();
List dataList = (List) result. getDataList();
if(dataList==null) {
Fx.log.info("query exception");
}
List idList = Lists. newArrayList();
//Loop through the query results and add all Ids to objectIds
for (int i=0;i<dataList. size();i++) {
Map map =(Map<String, Object>)(dataList. get(i));
Fx.log.info(map.get("name").toString());
idList.add(map.get("_id").toString());
}
Fx.log.info(idList);
//return id
return idList;
}
/**
* Function debugging method
* @param context function context
* @param args function parameters
*/
public void debug(FunctionContext context, Map<String, Object> args) {
execute(context, args);
}
}
3. The return type is RangeRule
Specify the default business type when selecting and creating new lookup association fields.
Function writing template:
Groovy:
QueryTemplate template = QueryTemplate. AND(
["name":Operator.LIKE("test")]
)
RangeRule rangeRule = RangeRule. builder()
.queryTemplate(template)
.recordType("record_cbxZ8__c") // This business type is used by default when searching for new associations
.build()
return rangeRule
Java:
import java.util.List;
import java.util.Map;
public class RangeRuleCode iimplements IRangeRuleAction {
/**
* The operation method of the range rule function (rangeRule) function
*/
@Override
public RangeRule execute(FunctionContext context, Map<String, Object> args) {
//Construct QueryTemplate
QueryTemplate template1 = QueryTemplate. AND(
Maps.of("name", QueryOperator.EQ("test")),
Maps.of("field_g7Zeh__c", QueryOperator.EQ("Test a single line of text"))
);
//construct RangeRule
RangeRule rangeRule = RangeRule. builder()
.queryTemplate(template1)
.recordType("record_cbxZ8__c") // This business type is used by default when searching for new associations
.build();
return rangeRule;
}
}